Linux operating system provides an outstanding environment for programming with its rich set of language and development tools. C is one of the most popular system programming languages to use in conjunction with Linux, in part because the operating system itself is written mostly in C. Using these languages, programmers can easily access system services using function libraries and system calls. Also, a variety of helpful tools can facilitate the development and maintenance of programs. In programming utilities, we discuss how to compile and link C programs, debug C programs using gdb, and make utility and system calls. The make utility helps you keep track of which program modules have been updated and helps to ensure that you use the latest versions of all program modules when you compile a program.
Compiling and Linking a C/C ++ Program
The GCC command is used to invoke GCC. GNU CC is a combined C and C ++ compiler so the gcc command also can compile C ++ source files. GCC uses the file extension to determine whether a file is C or C ++, C files have a lowercase e extension where as C ++ files end with c or cpp. To compile tabs.c and create an executable file named a.out gives the following command:
$ gcc tabs.c
The gcc utility calls the C preprocessor, the C compiler, the assembler, and the linker. The below given figure image shows these four components of the compilation process. The C preprocessor expands macro definitions and includes header files. The compilation phase creates assembly language code corresponding to the instructions in the source file. Then the assembler creates machine-readable object code. One object file is created for each source file. Each object file has the same name as the source file, except that the c extension is replaced with a .o. The preceding example creates a single object file named tabs.o. After successfully completing all phases of the compilation process for a program, the C compiler creates the executable file and then removes any .o files.
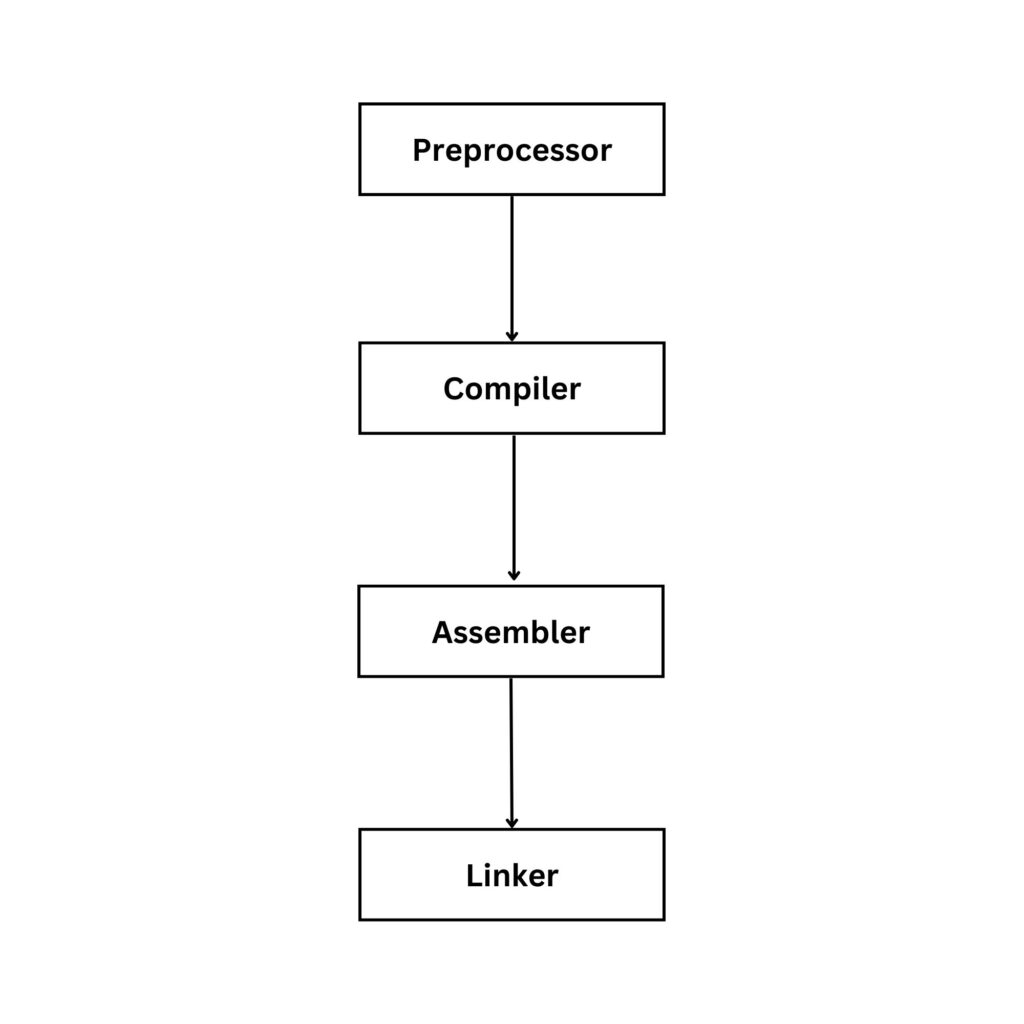
During the final phase of the compilation process, the linker searches specified libraries for functions, that the program uses and combines object modules for those functions with the program’s object modules. By default the C compiler links the standard C library libc.so (usually found in/lib), which contains functions that handle input and output and provides many other general-purpose capabilities.
If you want the linker to search other libraries, you must use the -1 (lowercase “1”) option to specify the libraries on the command line. Unlike most options for Linux system utilities, the – 1 option does not come before all filenames on the command line but usually appears after the filenames of all modules that it applies to. In the next example, the C compiler searches the math library libm.so (usually found in/lib):
$ gcc calc.c -1m
The -1 option uses abbreviations for library names, appending the letter following -1 to lib and adding a .so or .a extension. The m in the example stands for libm.so. Using the same naming mechanism, you can have a graphics library named libgraphics.a, which can be linked with the following command:
$ gcc pgm.c – 1graphics
When you use this convention to name libraries, gcc knows to search for them in/usr/lib and lib. You can have gcc also search other directories by using the -L option:
$ gcc pgm.c – L. – L/usr/X11R6/lib – 1graphics
The preceding command causes gcc to search for the library file libgraphics.a in the working directory and in /usr/X11R6/lib before searching /usr/lib and /lib. As the last step of the compilation process, the linker creates an executable file named a.out unless you specify a different filename with the -o option. Object files are deleted after the executable file is created. Although the gcc command can compile a C++ file, that command doesn’t automatically link with various class libraries that c++ programs typically require. Compiling and linking a C++ program by using the file g++ command is easy because it runs gcc with appropriate options.
If we want to compile the following simple C++ program stored in a file named hello C.
#include <iostream>
int main ()
{
using namespace std;
cout <<“hello from linux”<<endl;
}
To compile and the link this program into an executable program named hello, use this command:
g++ -o hello hello.c
The command create the hello executable, which we can run as follows
:/hello
The program displays the following output:
hello from Linux
make Utility
Make is a utility for managing large projects that are comprised of several source modules and libraries. For example, let’s say you have a project comprising 100 files. You go and change 3 source modules on Monday, then 2 more on Tuesday, then 4 more on Wednesday Now, it’s Thursday, and you need to re-compile your project, and you don’t recall which modules you had changed. Make can help by eliminating the need for you to keep track of the changed files.
First, make is a utility, but it uses another text file to describe exactly how to build a project. The file it uses is a text file that you must set up and is normally called makefile. If you just type ‘make’ at a linux prompt, the utility will search for the file makefile, and build the project based on that. You can also use the -f option to specify an alternate filename, besides makefile. makefile.
The organization of a make file is one of ‘targets’, ‘dependencies’, and ‘build rules’. Let’s assume we have 3 source modules in our project, called hello.cxx, funca.exx, and funeb.cxx. All three are needed to create the executable file hello. An example make file would look like the following:
# Make comments start with a ‘#’
hello: hello.o funca.o funcb.o funcs.h
hello.o : hello.cxx funcs.h
g++ -c hello.cxx
funca.o : funca.cxx funcs.h
g++ -c funca.cxx
Funcb.o : funcb.cxx funcs.h
g++ -c funcb.cxx
hello : hello.o funca.o funcb.o
g++ -o hello hello.o funca.o funcb.o
At the top, below the comment, is the main build line. It states that the ‘target’ hello is dependant upon the hello.o, Funca.o, funcb.o, and funcs.h files. This is just to establish the files needed, and does not have a ‘build rule’ (it is a single line). Two lines down (a blank line separates sections of a make file) is an example of a ‘target’, ‘dependency’, and build rule. This line says that hello.o is dependant upon hello.cxx and funcs.h.
What makes does, is compare the time and date of the target, against it’s dependencies. If the target is older than any of its dependencies, then it invokes the ‘build rule’ which is on the line immediately below it and indented. The build rule here is ‘g++ -c hello.cxx‘, which will re-create the hello.o file.
Make continues checking all the files and dependencies, re-building only those modules needed. The end result of the above make file will be to re-create the hello executable, only re-compiling the modules needed. It’s worth noting that the example above is an extremely simplistic one. The make utility is used in lots of places in Linux and has many features that we have not, and will not, touched on in this document. It provides all sorts of macros, variable names, and default build rules.