In this HackerRank Diagonal Difference problem solution Given a square matrix, calculate the absolute difference between the sums of its diagonals.
For example, the square matrix arr is shown below:
1 2 3
4 5 6
9 8 9
The left-to-right diagonal = 1+5+9 = 15. The right to left diagonal = 3+5+9=17. Their absolute difference is |15-17| = 2.
Function description
Complete the diagonalDifference function in the editor below.
diagonalDifference takes the following parameter:
- int arr[n][m]: an array of integers
Return
- int: the absolute diagonal difference
Input Format
The first line contains a single integer, n, the number of rows and columns in the square matrix arr.
Each of the next n lines describes a row, arr[i], and consists of space-separated integers arr[i][j].
Constraints
-100 <= arr[i][j] <= 100
Output Format
Return the absolute difference between the sums of the matrix’s two diagonals as a single integer.
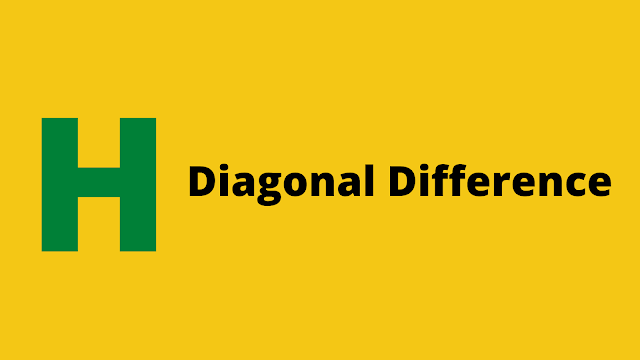
HackerRank diagonal difference problem solution in Python programming.
#!/bin/python3 import math import os import random import re import sys # # Complete the 'diagonalDifference' function below. # # The function is expected to return an INTEGER. # The function accepts 2D_INTEGER_ARRAY arr as parameter. # def diagonalDifference(arr): temp = 0 emp = 0 for i in range(0,len(arr)): temp = temp + arr[i][i] for j in range(0,len(arr)): emp = emp + arr[j][len(arr)-1-j] return abs(temp - emp) if __name__ == '__main__': fptr = open(os.environ['OUTPUT_PATH'], 'w') n = int(input().strip()) arr = [] for _ in range(n): arr.append(list(map(int, input().rstrip().split()))) result = diagonalDifference(arr) fptr.write(str(result) + 'n') fptr.close()
Problem solution in Java Programming.
import java.util.Scanner; public class DiagonalDifferernce { public static void main(String[] args) { Scanner in = new Scanner(System.in); int n ; int diag1 = 0 ; int diag2 = 0; n = Integer.parseInt(in.nextLine()); for(int i =0 ; i < n; i++){ String str[] = in.nextLine().split(" "); diag1 = diag1 + Integer.parseInt(str[i]); diag2 = diag2 + Integer.parseInt(str[n-1-i]); } int diagDiff = Math.abs(diag1 - diag2); System.out.println(diagDiff); } }
Problem solution in C++ programming.
#include <cmath> #include <cstdio> #include <vector> #include <iostream> #include <algorithm> using namespace std; int main() { /* Enter your code here. Read input from STDIN. Print output to STDOUT */ int matrix[100][100]; int i; cin>>i; for(int x=0;x<i;x++){ for(int y=0;y<i;y++){ cin>>matrix[x][y]; } } int diag1,diag2; diag1=0;diag2=0; for(int x=0;x<i;x++) { diag1=diag1+matrix[x][x]; } for(int x=i-1;x>-1;x--) { diag2=(diag2+matrix[i-x-1][x]); } int diff = diag1-diag2; if(diff<0){ cout<<-(diff); } else cout<<diff; return 0; }
Problem solution in C programming.
#include <stdio.h> #include <string.h> #include <math.h> #include <stdlib.h> int main() { int n,a[100][100],i,j,d1=0,d2=0,dif; scanf("%d",&n); for(i=0;i<n;i++) { for(j=0;j<n;j++) { scanf("%d",&a[i][j]); if(i==j) d1=d1+a[i][j]; if(i==(n-j-1)) d2=d2+a[i][j]; } } dif=abs(d1-d2); printf("%d",dif); return 0; }
Problem solution in JavaScript programming.
function processData(input) { var rightDia = 0; var leftDia = 0; var inputArray = input.split("n"); var squareSize = parseInt(inputArray.shift() - 1); for (var i = 0; i < inputArray.length; i++) { var row = inputArray[i].split(" "); rightDia += parseInt(row[i]); leftDia += parseInt(row[squareSize]); squareSize--; } console.log(Math.abs(rightDia - leftDia)); } process.stdin.resume(); process.stdin.setEncoding("ascii"); _input = ""; process.stdin.on("data", function (input) { _input += input; }); process.stdin.on("end", function () { processData(_input); });
please the solution in php
temp=0
emp=0
for i in range( 0 , len(arr)):
temp=temp+arr[i][i]
emp=emp+arr[i][len(arr)-1-i]
return abs(temp – emp)